In this blog post, I will walk through the journey to build a simple order fulfillment system using an Event-Driven Architecture (EDA). EDA is an architecture pattern designed to decouple and decentralize application components by allowing loosely couple services to communicate through asynchronously publishing and subscribing to events via an event router. EDA offers several advantages:
- Loose coupling: Components operate independently and their changes do not affect others as long as their interface remain the same. This enhances modularity and making it easier to add new services to the system.
- Scalability: Components can be added or modified easily to bring in new functionalities or scaled horizontally according to demands.
My simple order fulfillment system consists of the following processes:
- API endpoint that accepts order requests and publishes event to an event router via a Lambda function
- The event router is built using AWS EventBridge event bus that matches incoming requests’ location and routes them to target destinations for processing:
- Orders originating from the US will be routed to an API destination.
- Orders originating from Europe will be routed to a Step Functions workflow.
- All orders, regardless of their locations, will be routed to a Lambda Function to store the order details into a DynamoDB table and send back an order dispatch event to the event bus.
By following this approach, we can create a robust and flexible order fulfillment system capable of handling various requirements and scaling efficiently.
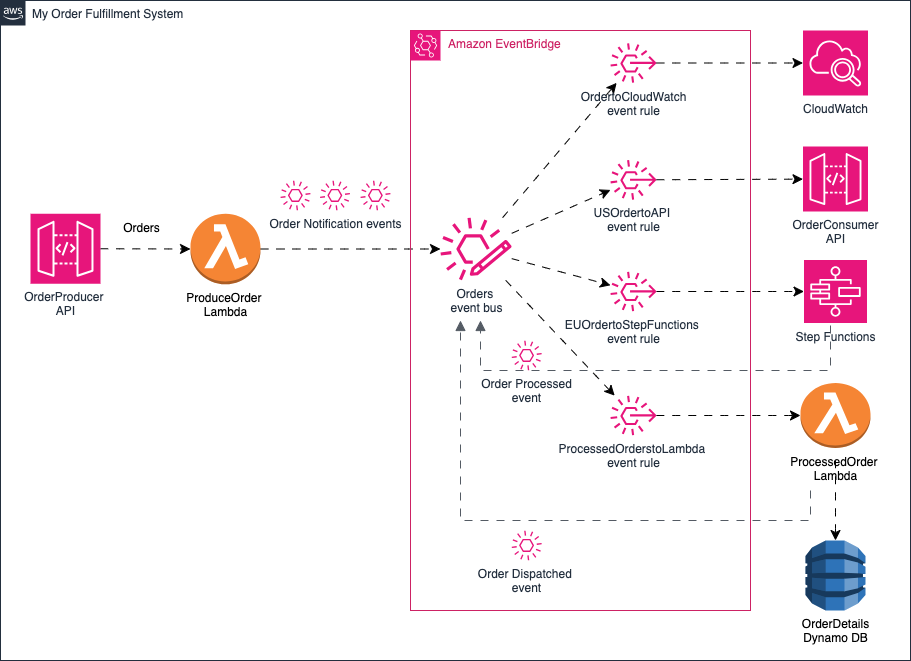
Objectives
- Create an Event Bus:
- Set up an AWS EventBridge event bus named Orders.
- Implement Event Rules:
- Create four event rules using AWS EventBridge:
- OrdertoCloudWatch
- USOrdertoAPI
- EUOrdertoStepFunctions
- ProcessedOrderstoLambda
- Create four event rules using AWS EventBridge:
- Create Lambda Functions:
- Develop two Lambda functions:
- ProduceOrder to publish events to the event bus.
- ProcessedOrder to handle events from the event bus.
- Develop two Lambda functions:
- Develop REST APIs:
- Set up two REST APIs:
- OrderProducer to accept order requests.
- OrderConsumer to process order requests.
- Set up two REST APIs:
- Implement a Workflow:
- Use AWS Step Functions to create a workflow for processing orders.
- Create a DynamoDB Table:
- Set up a DynamoDB table named OrderDetails to store order information.
- Establish IAM Policies:
- Create and configure IAM policies to grant necessary permissions to all services involved.
Step 1: Create Event Bus and CloudWatch Event Rule
Go to EventBridge console, select Event buses and click Create event bus
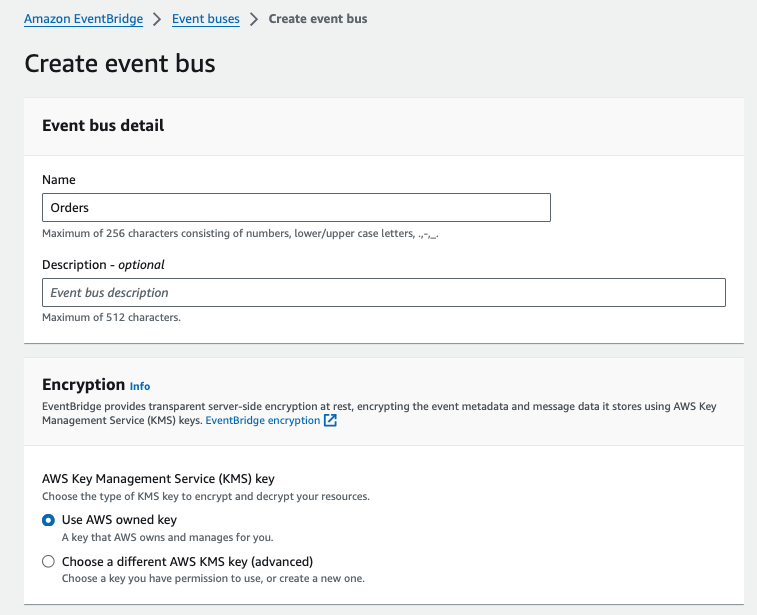
To easily test the event bus, I will create an event rule that act as a ‘catch-all’ for every event that passes to the event bus and writes it to a CloudWatch log.
Select the newly created Orders event bus and click Create rule:
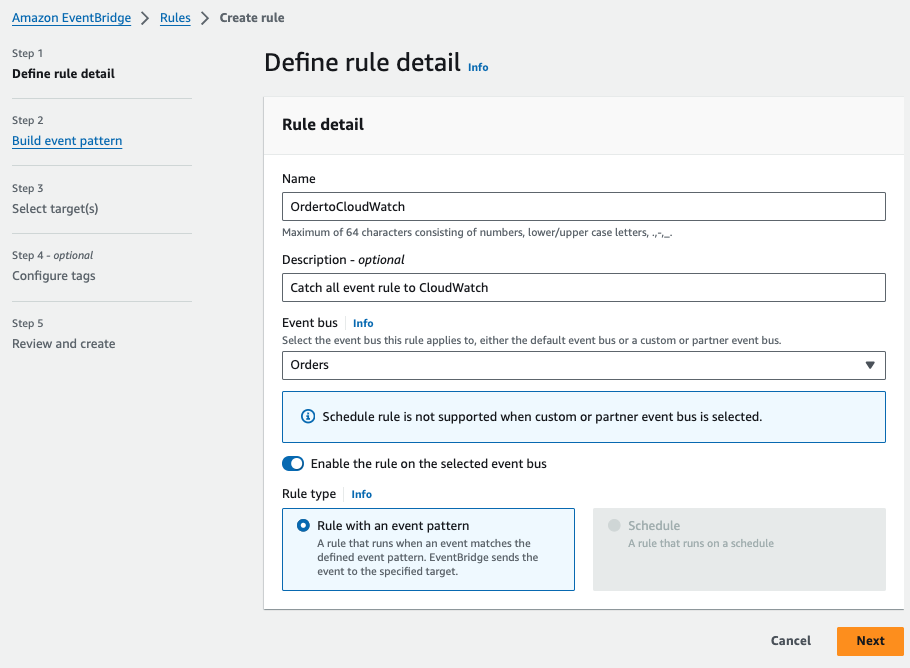
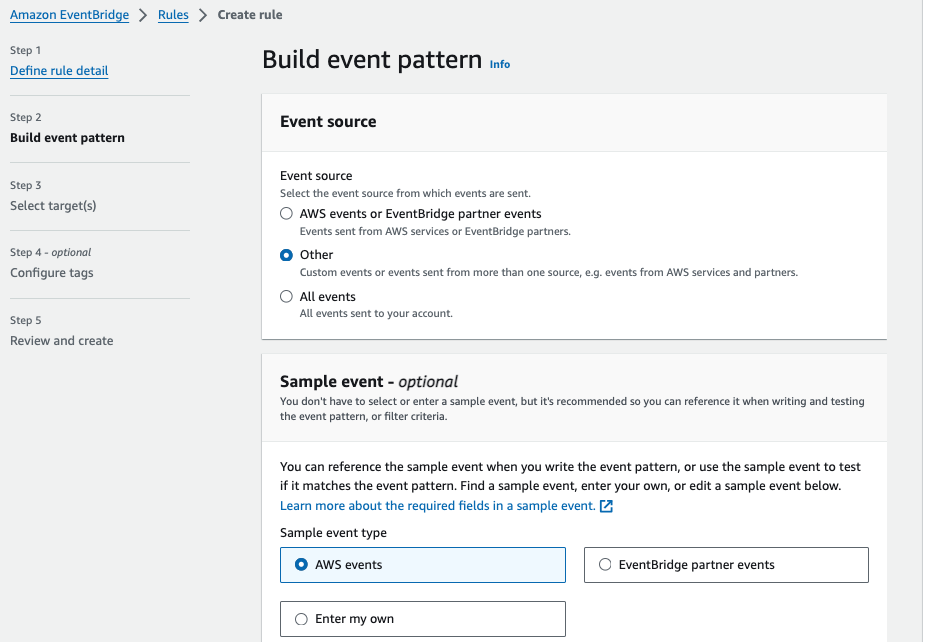
In the Event pattern, all events that come from source “com.aws.order” will be written to the CloudWatch log:
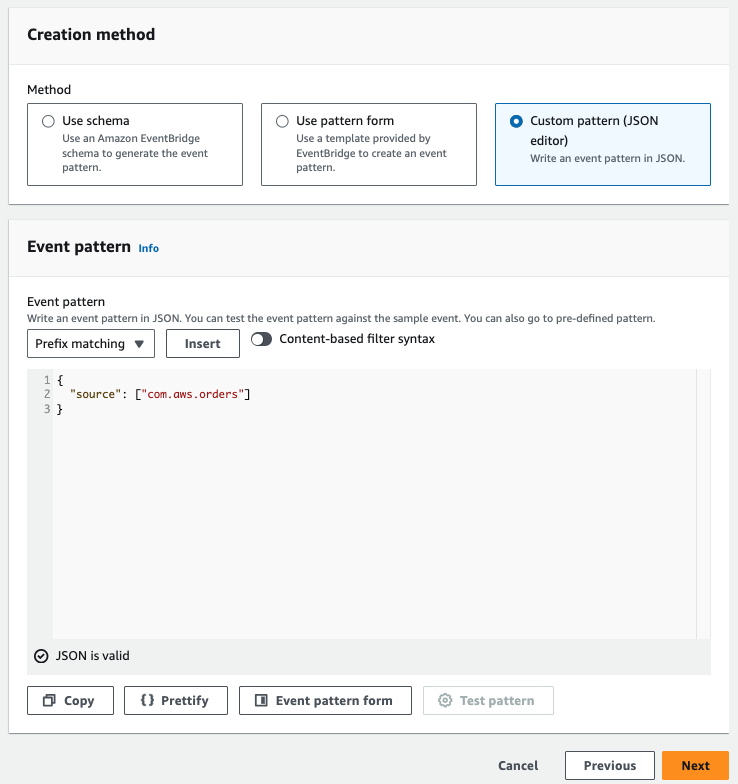
Select CloudWatch log group as the target for the event rule and name the log group suffix as /aws/events/orders
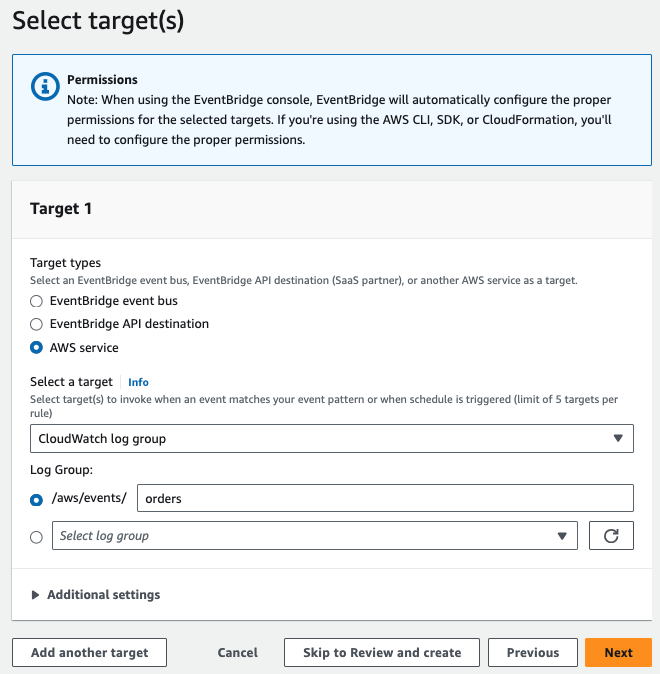
Now, every event sent to the Orders event bus will be logged to CloudWatch, making it easier to test and verify the events. To see the action, select Orders and click Send events with the following JSON payload:
Event Source: com.aws.orders
Detail Type: Order Notification
Event detail:
{
"category": "pencil",
"value": 200,
"location": "ca-central"
}
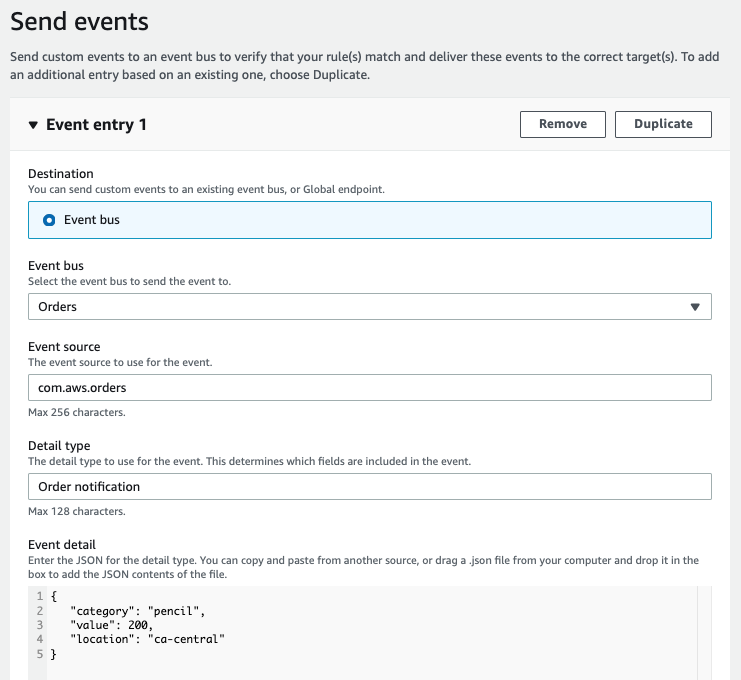
Open CloudWatch console, choose the log group /aws/events/orders and select the last log stream to verify that the event has been received by the event bus.
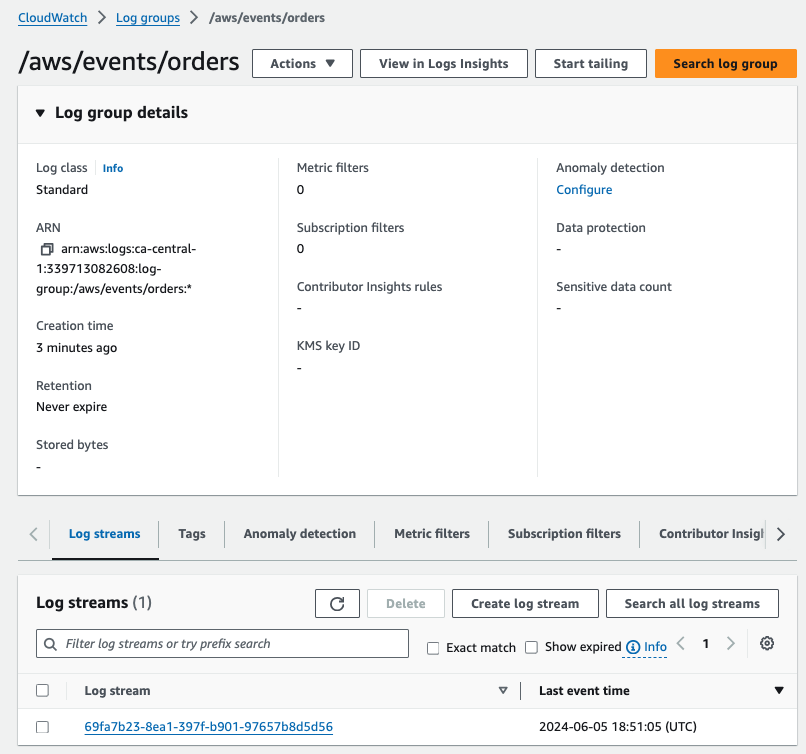
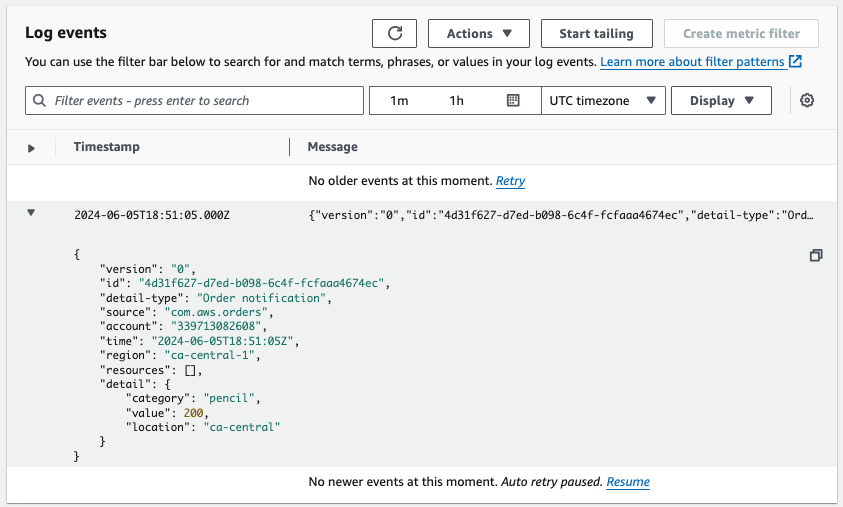
By following these steps, you can confirm that your ‘catch-all’ rule is working and that events are being properly logged to CloudWatch.
Step 2: Create Producer REST API and Lambda Function
I will create a Lambda function as a event producer to publish new order request to the event bus. Go to Lambda Function console and select Create function. The function reads an order details (location, category, value) from its parameters and publish the details to the Orders event bus.
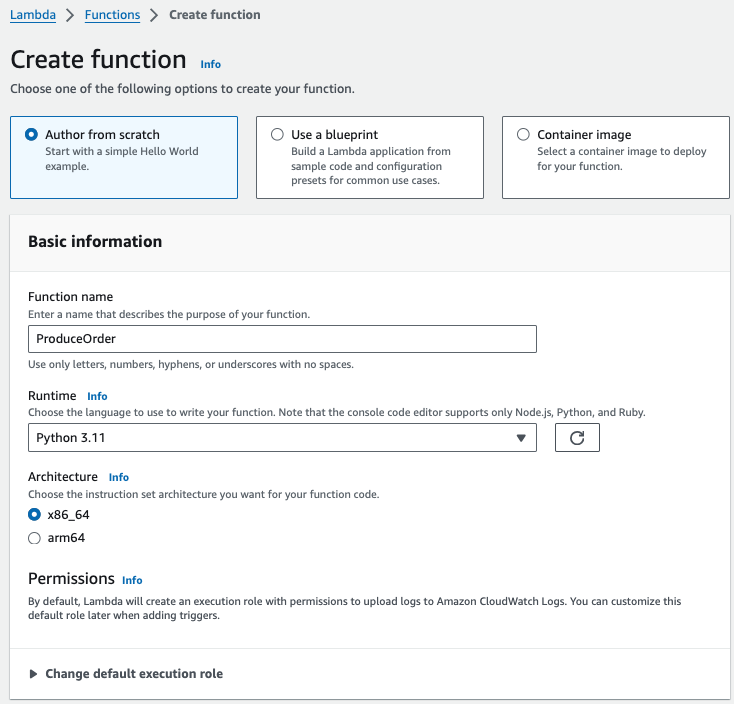
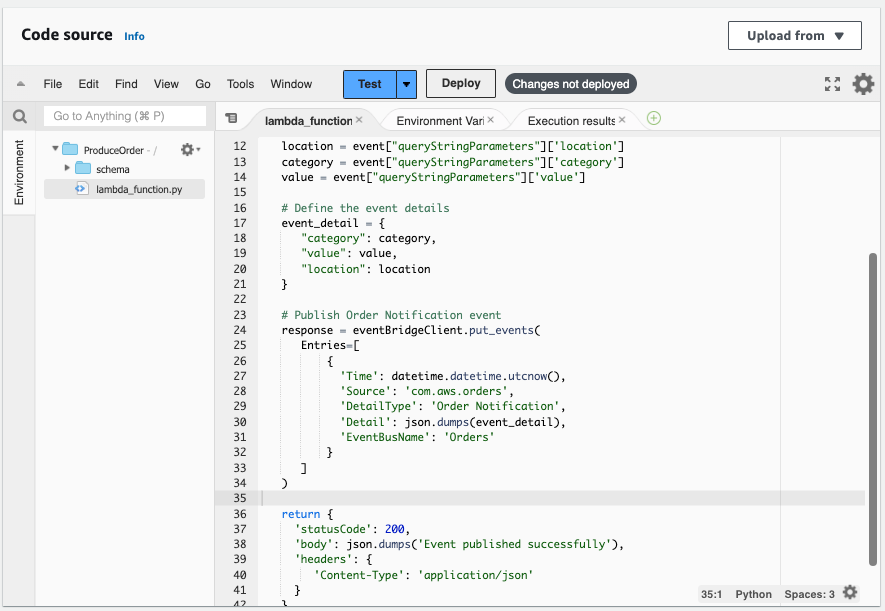
I will use API to simulate external parties making order requests and invoke my Lambda function to publish the order to the event bus. Go to API Gateway and click Create REST API.
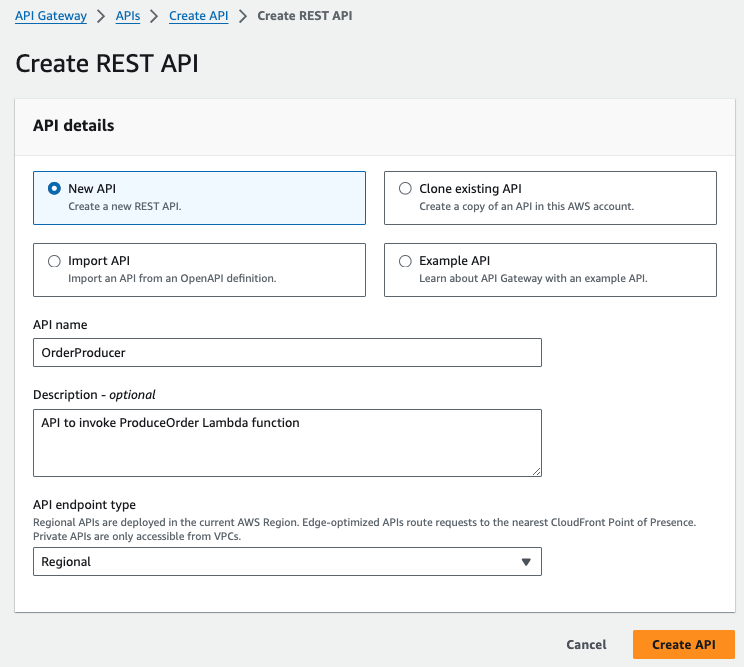
Select the ProduceOrder Lambda Function for integration.
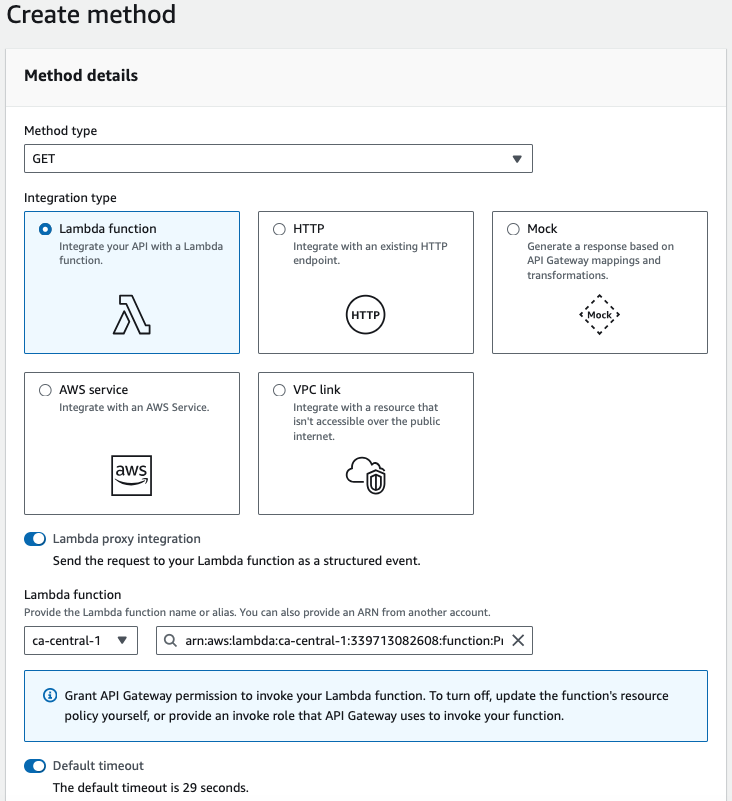
Select Deploy API and create a new stage for deployment.
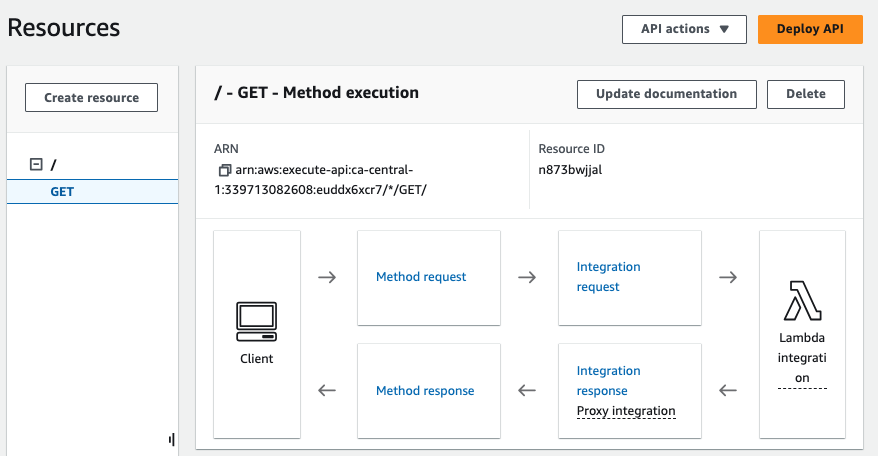
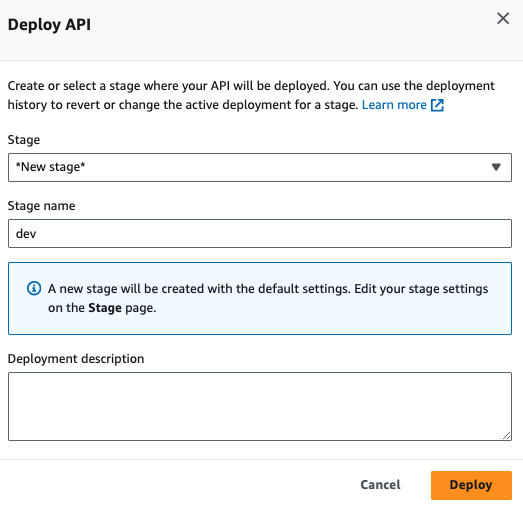
After deploying the API, we can find the Invoke URL in Stages. To test the API, simply open the URL from the browser.
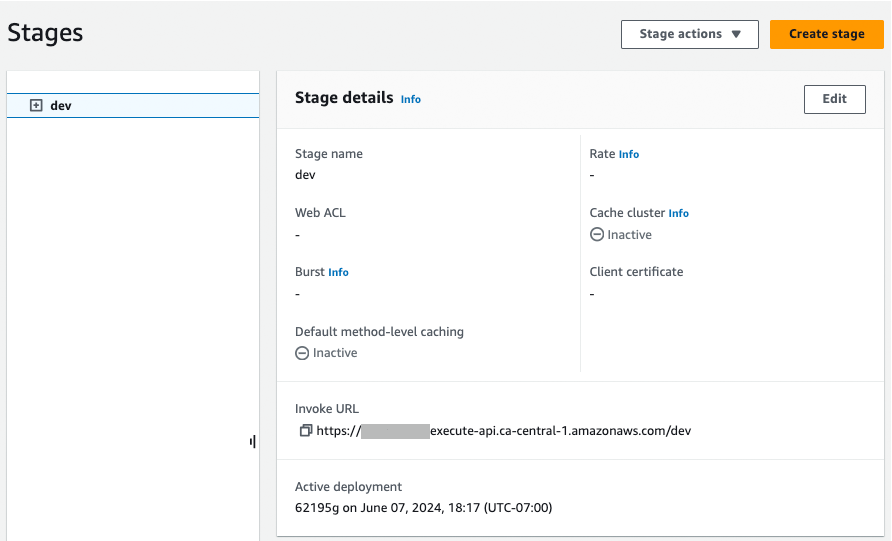
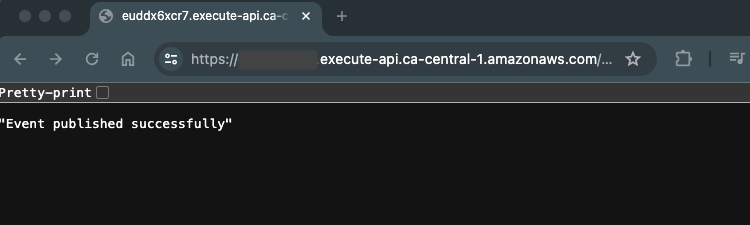
By following these steps, you can simulate external parties making order requests through the API, which will invoke the Lambda function to publish the order to the event bus.
Step 3: Event Rule to Route Orders to API Gateway
EventBridge event rules use event patterns to select events and route them to targets. Events in AWS EventBridge are presented as JSON objects with following structure:
{
"version": "0",
"id": "6a7e8feb-b491-4cf7-a9f1-bf3703467718",
"detail-type": "Order Notification",
"source": "com.aws.orders",
"account": "111111111111",
"time": "2024-06-08T18:43:48Z",
"region": "ca-central-1",
"resources": [],
"detail": {
"category": "supplies",
"value": 100,
"location": "us-east",
}
}
I will create my first event rule to route orders with location matching ‘us-east’ to an API destination. My event pattern looks like this:
{
"source": ["com.aws.orders"],
"detail": {
"location": ["us-east"]
}
}
First I will setup the API destination by creating a dummy REST API. Go to API Gateway console and choose Create API.
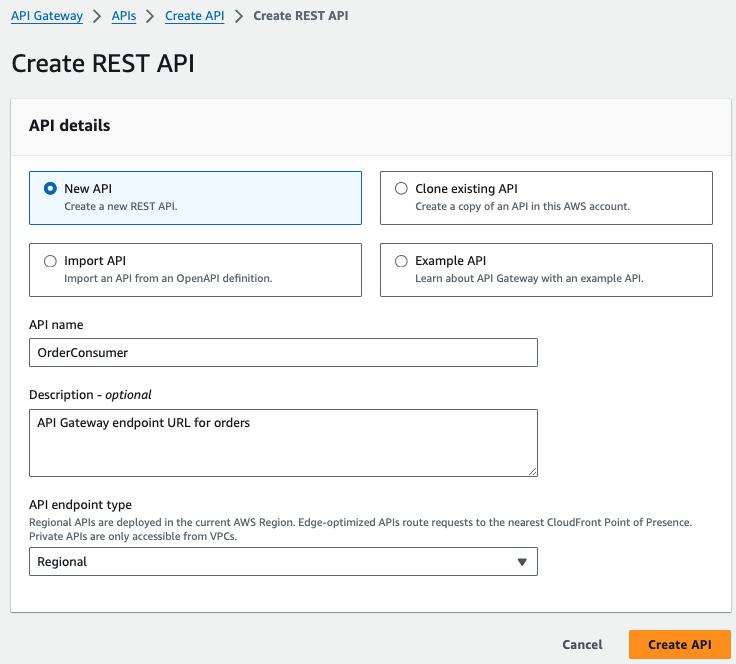
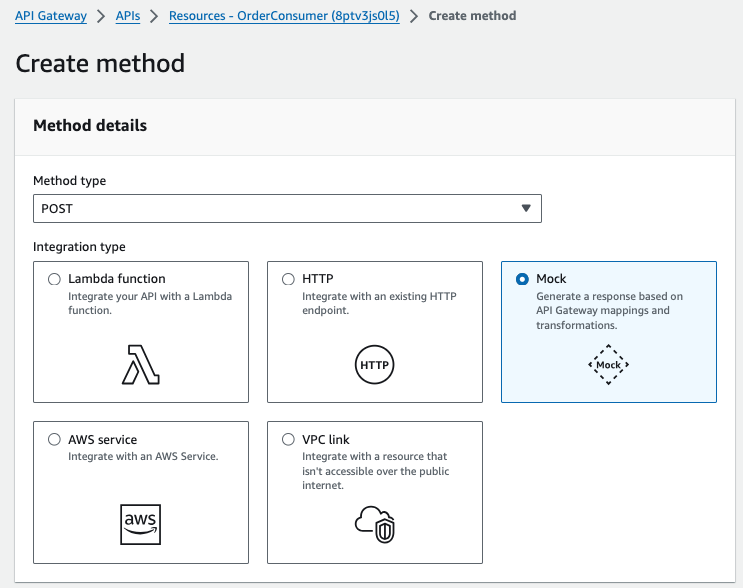
Deploy the API to obtain the API destination endpoint (e.g., https://<app-id>.execute-api.<region>.amazonaws.com/dev).
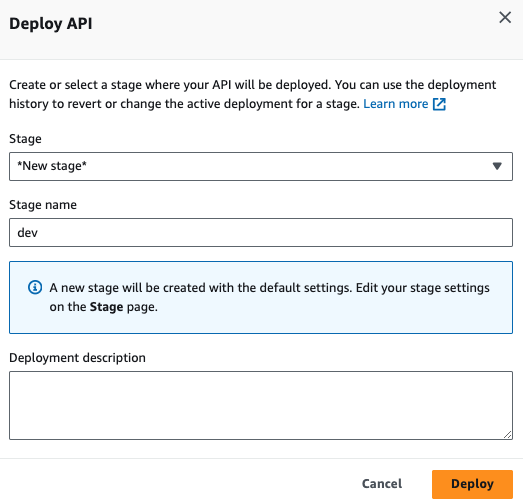
Now we create the event rule, select EventBridge Rules and click on Create rule
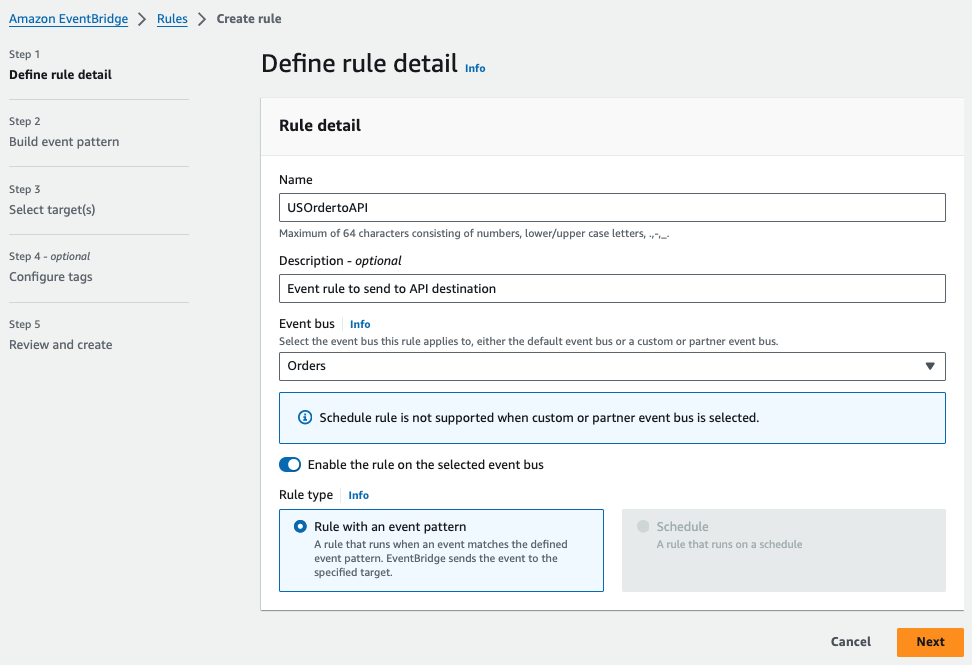
Copy and paste the above event pattern and select Next.
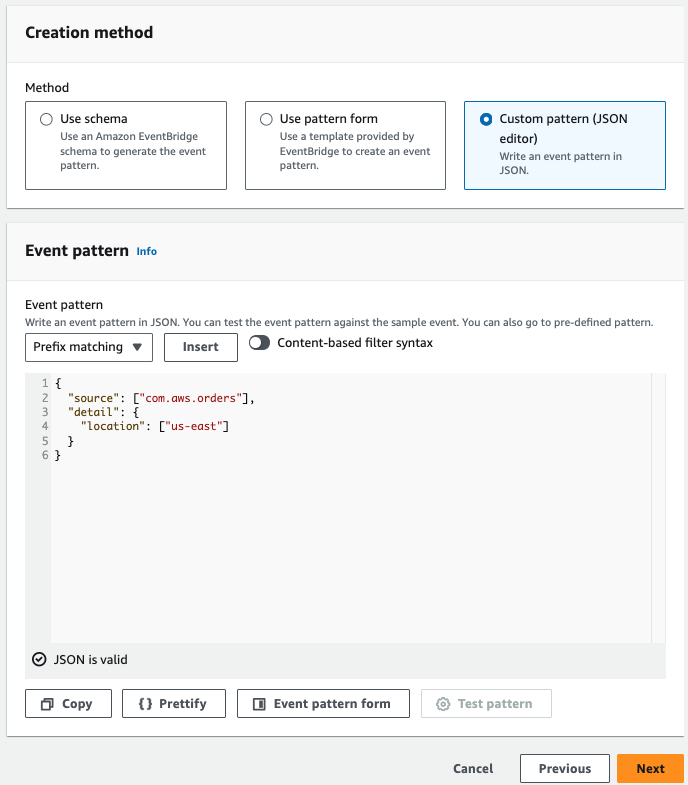
To configure the event rule to route to an API destination, we need to create a new API destination. Under API destination, choose Create a new API destination with the following:
- API destination endpoint: <https://<app-id>.execute-api.<region>.amazonaws.com/dev>
- HTTP method: POST
- Connection type: Create a new connection
- Authorization type: Basic (Username/Password)
- Username: myUsername
- Password: myPassword
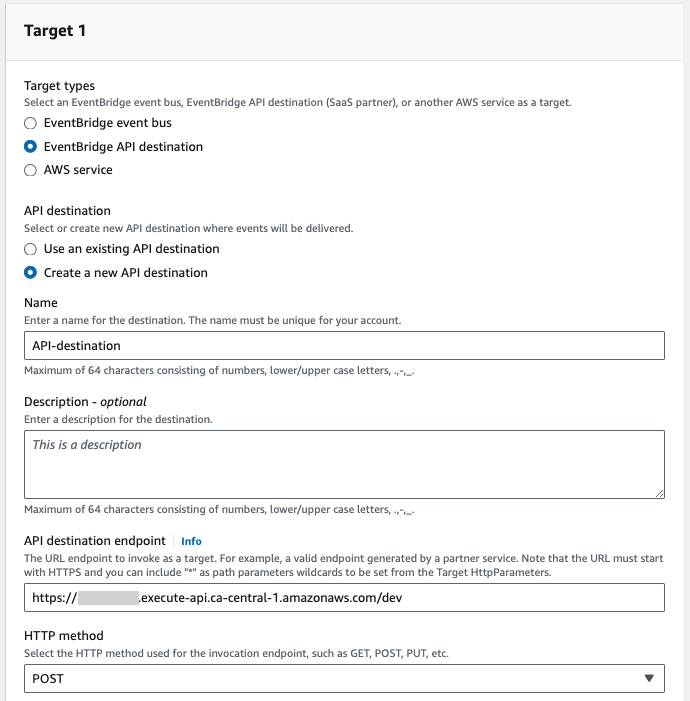
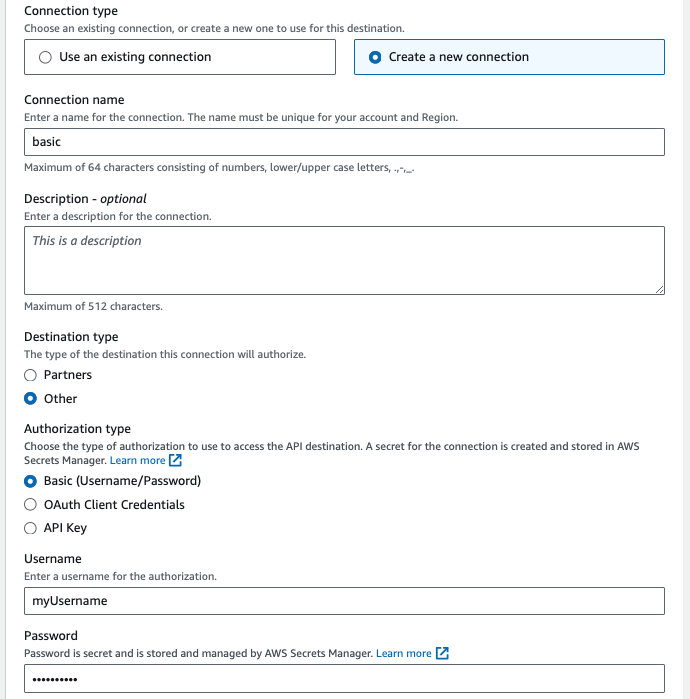
To verify the event rule, I can simply call our Producer API with the following parameters:
https://xxx.execute-api.ca-central-1.amazonaws.com/dev?location=us-east&category=office-supplies&value=200
To see the log, open CloudWatch Log Group console and select the Log group API-Gateway-Execution-Logs prefix. The log event shows the API responds successfully with a 200 status.
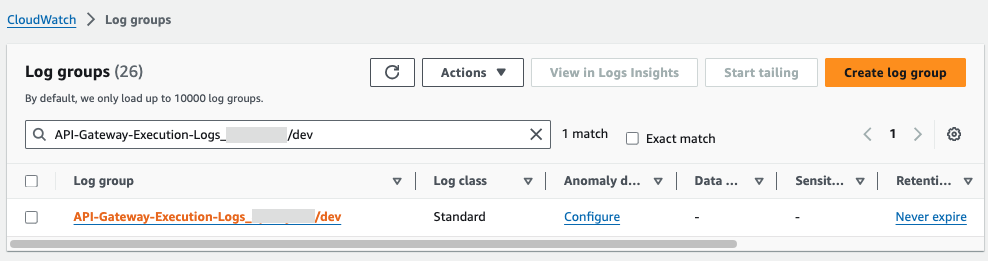

Now, any order event with the location set to us-east will be routed to the API Gateway endpoint. This setup ensures that orders from the specified region are correctly processed by the designated API.
Thank you for reading my post. Stay tuned for Part 2, where I’ll cover the remaining implementation steps.