When managing AWS resources, it’s common to start with resources created manually or by other tools. As you begin adopting Infrastructure as Code (IaC) with Terraform, importing these existing resources becomes necessary. In this blog, we’ll explore how to import AWS Systems Manager State Manager association into Terraform, and then how to update the EC2 instance IDs within the Terraform-managed resource.
Prerequisite
To use Terraform you will need to install a binary package for your platform (e.g. macOS or Windows). For macOS, you can install the Homebrew packages using the following commands:
brew tap hashicorp/tap
brew install hashicorp/tap/terraform
Step 1: Importing AWS Systems Manager State Manager Association
AWS Systems Manager State Manager is a powerful tool that helps manage the configuration of your instances. To manage existing State Manager associations with Terraform, you’ll first need to import them.
First, create a Terraform configuration file (e.g. ssm.tf) with a place holder for your SSM association. You don’t need to fill in all the arguments, just create a basic structure. Here’s an example:
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "5.63.1"
}
}
}
# Configure the AWS Provider
provider "aws" {
region = "us-west-2"
}
resource "aws_ssm_association" "myEC2FreeSpace" {
name = "myEC2FreeSpace"
}
Before any import, you need to initialize your Terraform working directory. It downloads the necessary provider plugins, setup backend, and prepares the environment for running other Terraform commands.
terraform init
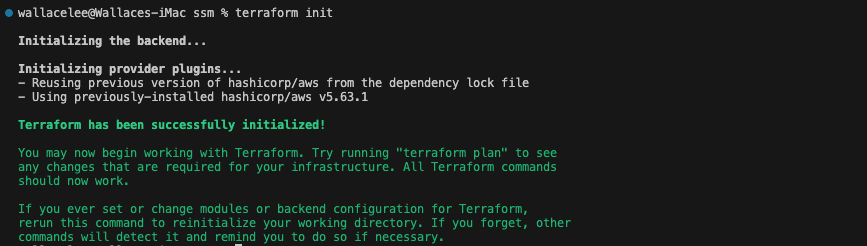
Use the terraform import
command to import the existing association into Terraform:
terraform import aws_ssm_association.myEC2FreeSpace <association-id>
Replace <association-id>
with the actual ID of the State Manager association. After importing, Terraform will manage this resource, and you can use terraform plan
to see the current configuration.

After importing, Terraform will now have the association in its state, but the resource block you initially created will likely be incomplete. Run the following command to see what Terraform detects as different between your configuration and the actual state:
terraform plan
Terraform will show the differences, and you should update your aws_ssm_association
resource block in your ssm.tf
file to match the actual state configuration. This may include adding parameters, targets, document version, etc.
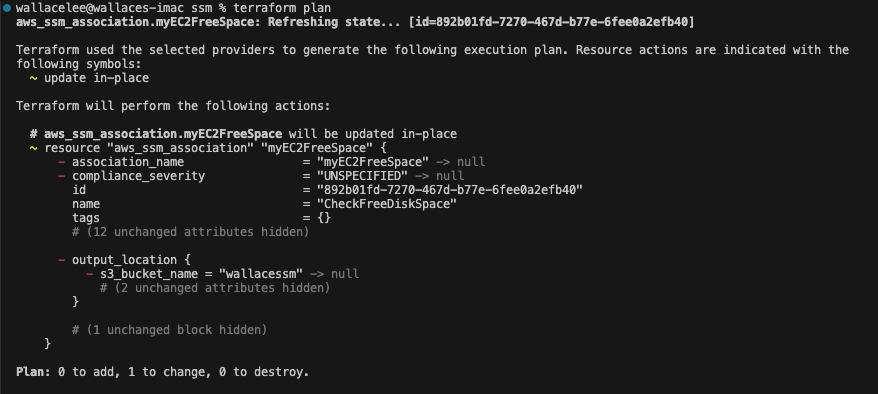
Run the show command and copy the current terraform state to the configuration for editing.
terraform show
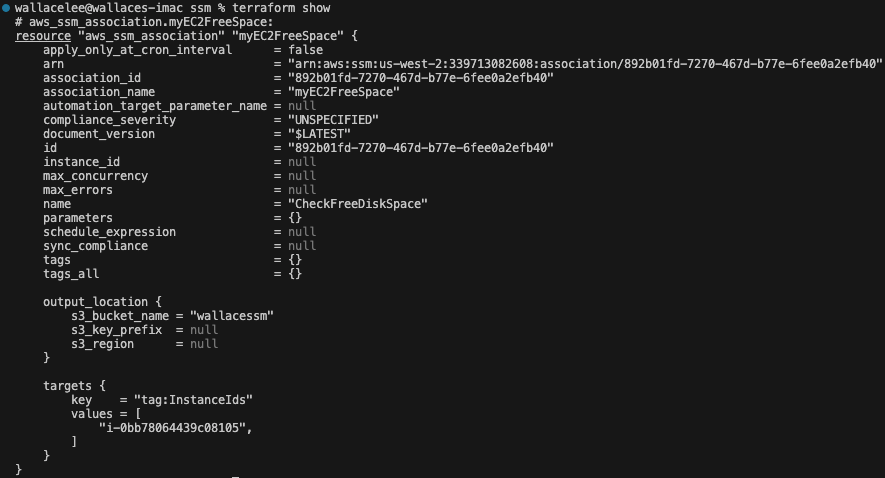
Edit the configuration until it matches the existing state.
#SSM State Manager Association
resource "aws_ssm_association" "myEC2FreeSpace" {
association_name = "myEC2FreeSpace"
document_version = "$LATEST"
name = "CheckFreeDiskSpace"
schedule_expression = "cron(0 */30 * * * ? *)"
compliance_severity = "UNSPECIFIED"
tags = {}
targets {
key = "tag:InstanceIds"
values = [
"i-0abcd1234efgh5678",
]
}
}

Step 2: Modify EC2 Instance ID in State Manager Association
To update the instance ID in the State Manager association using Terraform, modify your Terraform configuration:
#SSM State Manager Association
resource "aws_ssm_association" "myEC2FreeSpace" {
association_name = "myEC2FreeSpace"
document_version = "$LATEST"
name = "CheckFreeDiskSpace"
schedule_expression = "cron(0 */30 * * * ? *)"
compliance_severity = "UNSPECIFIED"
tags = {}
targets {
key = "tag:InstanceIds"
values = [
"i-0abcd1234efgh5678",
]
}
}
Replace "i-0abcd1234efgh5678"
with the new EC2 instance ID.
Once you’ve made the necessary updates to your configurations, verify the changes with:
terraform plan
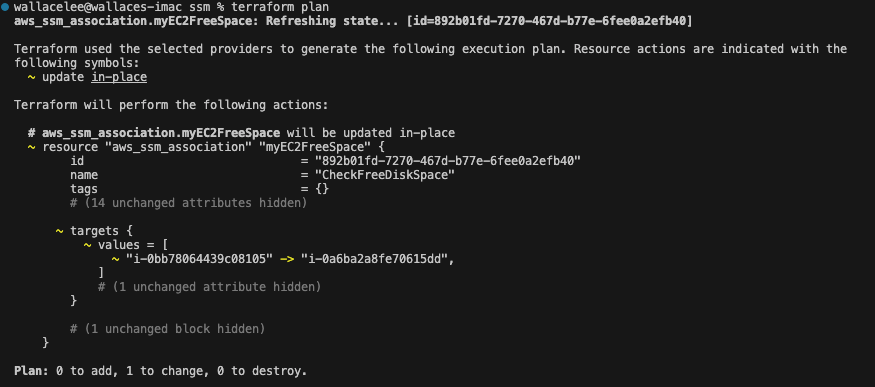
Apply the changes with:
terraform apply
This command will update the existing State Manager association with the new EC2 instance ID.
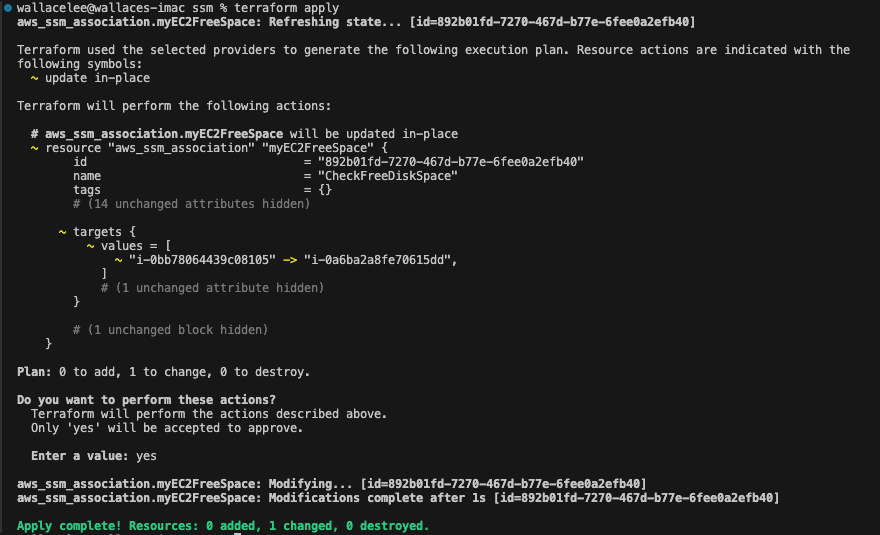
Final Thoughts
Managing AWS resources using Iac offers several key benefits. It greatly reduces the time required to deploy infrastructure and allows you to track changes in your configurations through version control, making it easier to maintain and audit. Once you have your environment setup using Terraform, you can easily replicate another (e.g. staging, production) by applying the same configurations, ensuring consistency across different environments.
Thank you for reading and I hope you like my blog.